About TimeGPT
Get started with our QuickStart guide, walk through tutorials on the different capabilities, and learn from real-world use cases in our documentation.
Overview
Nixtla’s TimeGPT is a generative pre-trained forecasting model for time series data. TimeGPT can produce accurate forecasts for new time series without training, using only historical values as inputs. TimeGPT can be used across a plethora of tasks including demand forecasting, anomaly detection, financial forecasting, and more.
The TimeGPT model “reads” time series data much like the way humans read a sentence – from left to right. It looks at windows of past data, which we can think of as “tokens”, and predicts what comes next. This prediction is based on patterns the model identifies in past data and extrapolates into the future.
The API provides an interface to TimeGPT, allowing users to leverage its forecasting capabilities to predict future events. TimeGPT can also be used for other time series-related tasks, such as what-if scenarios, anomaly detection, and more.
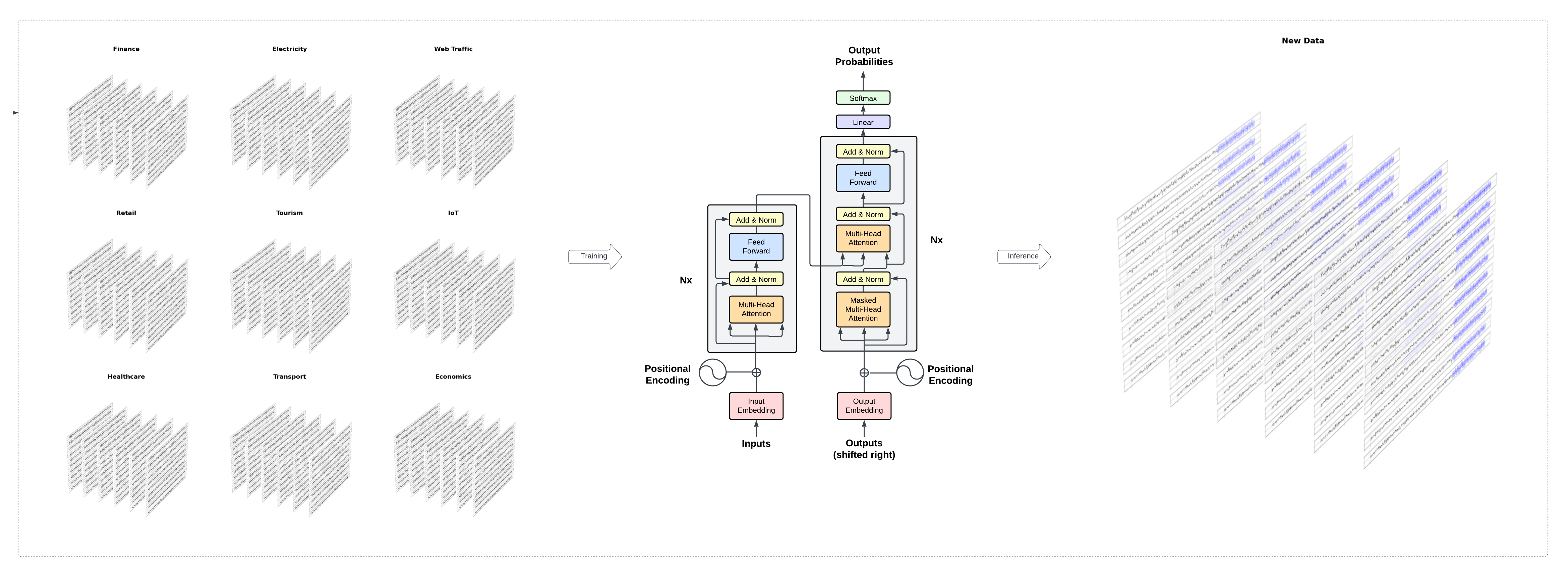
Explore TimeGPT API
The capabilties section highlight all of the model’s functionalities with short code snippets for easy and quick experimentation.
For more detailed tutorials, check our in-depth guides covering all the steps required to use TimeGPT to its full potential.
Make sure to also check our uses cases to see TimeGPT applied to real-life scenarios.